Abstract
Have you ever heard of the term "polymorphism" in Java programming? The first time i heard of it, my mind instantly went to my days playing Hearthstone. If you know you know... Nonetheless, If you're intrigued by the idea of flexible and dynamic code, then you're in for a treat. In this blog, we'll delve into the fascinating world of Java polymorphism, exploring how they bring versatility and power to your programs. To make things more concrete, we'll examine some example code that demonstrates both compile-time and runtime polymorphism. So, let's jump right in!
The Concept of Polymorphism:
Before we dive into the code, let's briefly understand what polymorphism is all about. In Java, polymorphism refers to the ability of an object to take on different forms or behaviors depending on the context. It allows you to write more flexible and reusable code, making your programs easier to maintain and extend.
Compile-Time Polymorphism:
Let's start by exploring compile-time polymorphism. The code snippet below showcases this type of polymorphism:
JAVASCRIPTpublic class Polymorphisms { Polymorphisms() { System.out.println("Polymorphisms()"); } void Describe(int input) { System.out.println("I'm a compile-time polymorphism that takes an int"); } void Describe(String input) { System.out.println("I'm a compile-time polymorphism that takes a String"); } }
In this example, the class Polymorphisms contains two methods with the same name, Describe, but with different parameter types (int and String). This is known as method overloading, a form of compile-time polymorphism. At compile time, the Java compiler determines which version of the method to invoke based on the arguments passed. So, when you call Describe(42), the compiler knows to execute the method that accepts an int, and when you call Describe("Hello"), it selects the method that takes a String.
Runtime Polymorphism:
JAVASCRIPTclass Runtime_Polymorphism extends Polymorphisms { Runtime_Polymorphism() { System.out.println("Runtime_Polymorphism()"); } @Override void Describe(int input) { System.out.println("I'm a runtime polymorphism!"); } }
In this case, the class Runtime_Polymorphism extends Polymorphisms and overrides the Describe method that accepts an int. When you create an object of type Runtime_Polymorphism and call the Describe method with an int argument, it executes the overridden method defined in the subclass. This is runtime polymorphism, also known as method overriding. At runtime, the JVM dynamically determines which implementation of the method to invoke based on the actual type of the object.
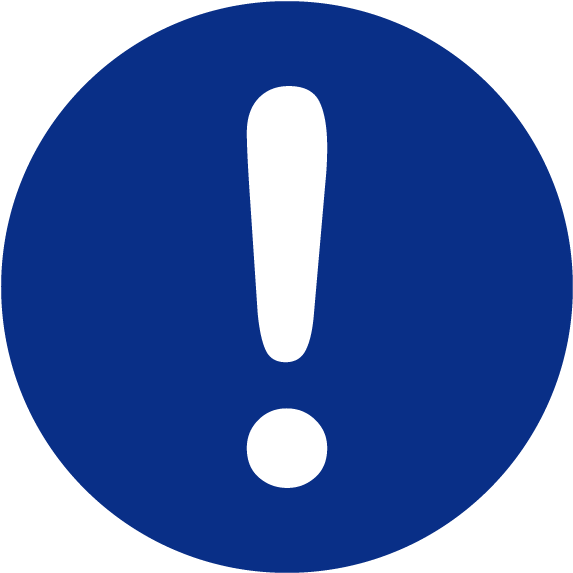
Both types of polymorphism's can be used in tandem! For example, you may want to have an abstract class with method overloading, but some child classes with method overriding.
Understanding the Magic:
Now that we've examined both compile-time and runtime polymorphism, let's reflect on their significance. Compile-time polymorphism, achieved through method overloading, allows you to define multiple methods with the same name but different parameter types. It provides flexibility and convenience by enabling you to use a single method name for similar operations that vary based on input types.
On the other hand, runtime polymorphism, accomplished through method overriding, lets you define specialized behavior for methods inherited from a super class. It promotes code extensibility and customization by allowing subclasses to provide their own implementation while adhering to the same method signature.
Performance Considerations:
While polymorphism brings flexibility and code elegance, it's important to consider performance implications when utilizing polymorphic code. In the case of compile-time polymorphism (method overloading), the Java compiler determines the appropriate method to invoke at compile time, resulting in efficient execution. However, keep in mind that excessive method overloading with a large number of parameters or complex method signatures can impact code readability and maintainability.
On the other hand, runtime polymorphism (method overriding) incurs a slight performance overhead due to dynamic method resolution at runtime. However, modern JVM optimizations mitigate this overhead, and the benefits of code extensibility and flexibility often outweigh the minimal performance impact. As always, it's crucial to strike a balance between code design and performance considerations to ensure optimal software performance.
Closing
Congratulations! You've now been poly-morphed into a 1-1 sheep! Yes another Hearthstone joke, I'm sorry. We've covered compile-time polymorphism using method overloading and runtime polymorphism through method overriding.
Embrace the power of polymorphism, and watch your Java programs become more versatile and adaptable.