If you've ever spent time fluffing around multiple css or scss files to find styles and font-sizes you've used on previous elements, this CSS variable best practice will help you!
Cascading Style Sheets (CSS) variables provide a powerful way to manage and maintain consistent design elements across a web application or website. In this article, I'll explore how to use CSS variables specifically for font sizes, showcasing their benefits, best practices, and how they contribute to design consistency. I'll also go into some examples of how we use it at Dear Developer.
The Basics - Setting CSS Variables
To set a CSS variable, you simple add a CSS property on an element in the format --var-key: value
CSS.example-element { --body-color: black; }
In doing so, we can now access this value in any element with the .example-element class, or more importantly, in any of this elements descendants.
CSS/* Directly using class */ .element-example { background-color: var(--body-color); } /* Indirectly via discendant inheritance */ .element-example .child { background-color: var(--body-color); }
It's exactly this functionality, variable inheritance in child nodes, that form the basis for globally scoped variables for consistency in our styles.
Using this information, and a common best practice of setting our variables in the :root of the document, we can now set globally scoped variables that we can use across all CSS files included after or within our styles.css file.
CSS:root { --body-dark: black; --body-light: #ECECEC; } .any-element.light { background-color: var(--body-light); } .any-element.dark { background-color: var(--body-dark); }
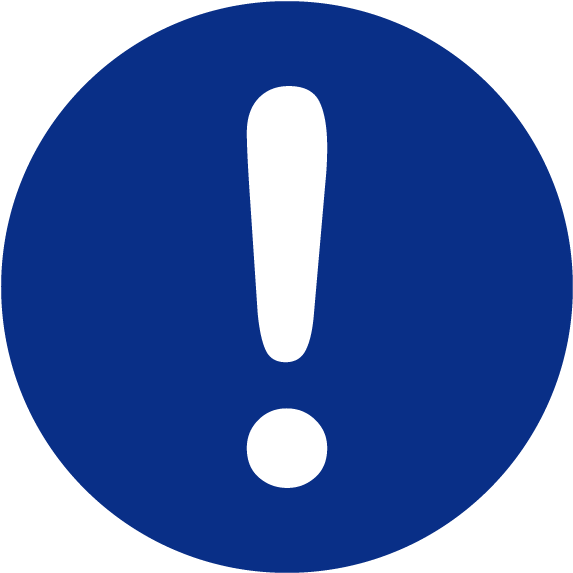
It's best practice to put variables in the :root, because they will become globally scoped and can be used everywhere.
An important thing to note about CSS variables, is that which value gets chosen works the same way as how values get chosen when using other CSS properties:
CSS:root { --font-bold: 500; } h1 { /* This variable will override the variable from the root */ --font-bold: 800; font-weight: var(--font-bold); }
Consistency and Scalability - Using Relative Units For Fonts
With the basics of using variables out of the way, lets jump into another best practice to level up your font-size usage. Using rem instead of px units when declaring font-sizes.
This practice aligns with the principles of consistency and responsiveness, contributing to a more polished and adaptable design.
Relative units, such as rem, offer dynamic and responsive font sizing compared to the fixed nature of px. Unlike px, which sets an absolute size, rem units are tied to the root element's font size. When you adjust the root font size, all other rem units in your stylesheet proportionally scale accordingly. This feature makes rem an ideal choice for creating designs that seamlessly adapt to different screen sizes and devices.
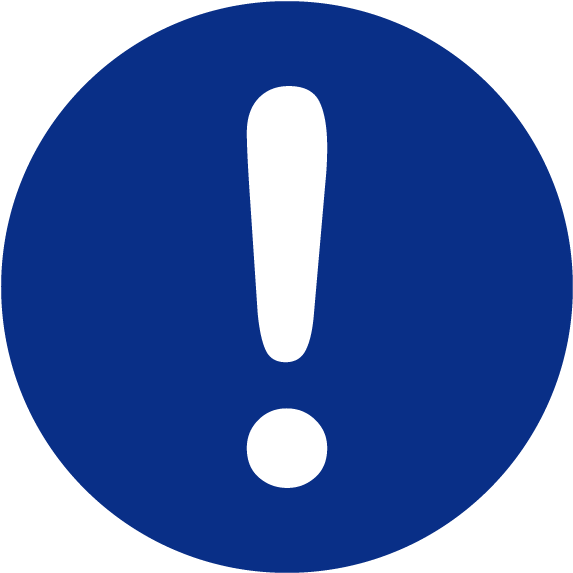
rem stands for root element, and the value of 1rem as normally set by browsers is: 16px.
Implementation
CSS:root { /* Dear Developer Example */ /* Font Size Design System */ --font-size-xsmall: 0.875rem; --font-size-small: 1rem; --font-size-medium: 1.125rem; --font-size-large: 1.56rem; --font-size-xlarge: 2rem; }
These values might initially look scary and confusing, but if you wish to swiftly convert them to equivalent pixel values within the context of the base scaling before accounting for user preferences:
0.875rem = 16*0.875 = 14px
1rem = 16*1 = 16px
1.125rem = 16*1.125 = 18px
A pivotal advantage of opting for rem units instead of px units lies in their synergy with user font-size preferences, as honored by modern browsers. These preferences often stem from factors like operating system scale settings.
By embracing rem, the web design seamlessly accommodates the browser's interpretation of a user's font-size choice. This translates into a coherent and anticipated scaling effect, ensuring that all typography retains a consistent and anticipated proportional increase. In essence, your design respects and harmonizes with user preferences, resulting in a dynamic and comfortable user experience.
Putting it all together
let's put these practices to work with a practical example. Imagine we're designing a sleek, responsive website, and we want to ensure consistency and adaptability in our design.
First, we'll start by setting our CSS variables in the :root of our document as explained earlier.
CSS:root { --main-font-size: 1rem; --secondary-font-size: 0.875rem; --primary-color: #3498db; /* Add more variables as needed */ }
Next, let's tackle font scalability. Instead of using fixed px units for font sizes, we'll use rem units. Remember, 1rem is typically equal to 16px, and this scaling factor is automatically applied by browsers.
CSSbody { font-size: var(--main-font-size); } h1 { font-size: calc(var(--main-font-size) * 2); } p { font-size: var(--secondary-font-size); }
In this example, our base font size is set to 1rem, which gives us 16px. Headers (h1) are twice the base size, and paragraphs (p) are slightly smaller. This consistent scaling ensures that our typography adapts gracefully across different devices and user preferences.
Incorporating CSS variables and using rem units for font sizes empowers us to create web designs that are not only visually appealing but also highly adaptable. The :root-scoped variables grant us a centralized control hub for our design elements, promoting consistency.
Meanwhile, rem units offer responsiveness by tying font sizes to the root element's size, accommodating user preferences effortlessly. This synergy between variables and relative units results in a harmonious user experience, where typography scales seamlessly and remains accessible to all users.